Let's assume I currently just have an enum containing the skills FireBall, Rage, Heal, and Melee as my ability system. I have a method that makes use of the currently chosen skill. When the space bar is pushed in the Update Method, the current ability is used.
The issue with this is that the more code I need to put to this one script, the more difficult an ability is. Really, there is no way to distinguish between these. If I wish to add more powers, equip more than one ability, or have varied abilities depending on which key is struck, that presents another issue. Things might soon spiral out of control.
Solution
Have the ability runner maintain their present field of abilities.
An interface with a Use method is referenced by this capability.
I'll then be able to have powers that come from the Ability Interface.
If I alter the names in this instance, what I simply did is apply the strategy pattern.
What is the Strategy Pattern exactly?
Encapsulating or wrapping up a behavior or algorithm in a separate class is the primary idea behind the strategy pattern. The ability to set actions or algorithms during runtime is an extra benefit! The strategy pattern may be precisely what you need to organize your code if it now has a tangle of if statements and switch statements that are trying to manage or modify behaviour. The strategy pattern states that a class's actions shouldn't be passed down through inheritance. Interfaces should be used to encapsulate them instead. The Open but Closed SOLID concept, which suggests that classes should be open for extension but closed for modification, is consistent with this. This design is excellent for developing Ability systems, AI behaviors, or weapon systems.
Definitions
- Strategy — Declares an interface common to all supported algorithms. Context uses this interface to call the algorithm defined by a Concrete Strategy.
- Concrete Strategy — Implements the algorithm using the Strategy interface.
- Context — Is configured with a Concrete Strategy object. Maintains a reference to a Strategy object. May define an interface that lets Strategy access its data.
Implementation
IAbility
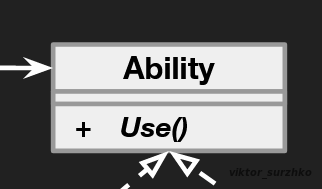
Making the Ability Interface is the first stage.
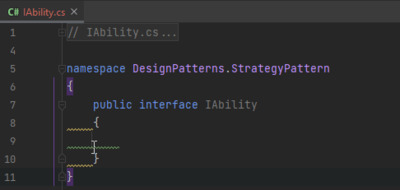
Converting Ability Runner will employ the new user interface
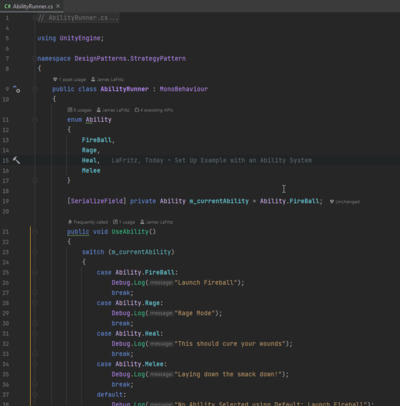
Abilities
Each ability must then be created by implementing the IAbility interface.
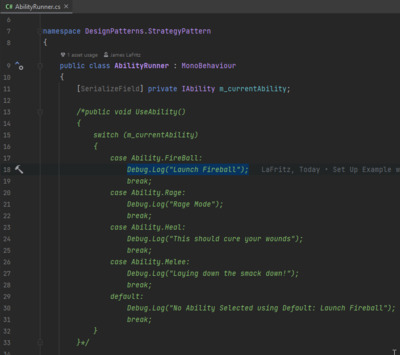
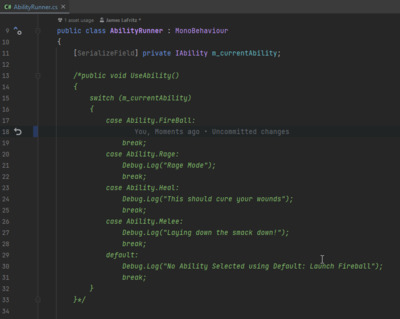
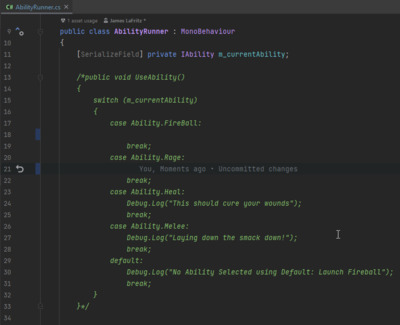
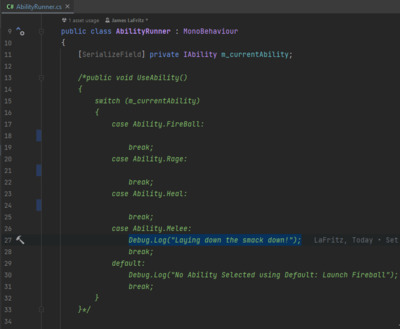
Using abilities
None of my recently developed skills are currently used by the ability runner. I need a mechanism to modify the ability runner's current ability; however, because Unity does not serialize interfaces (Built In), I am unable to assign this field in the Inspector at the moment.
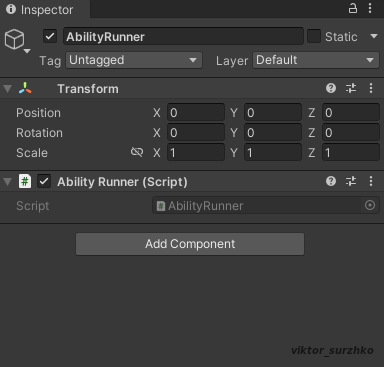
There are several methods to put this into practice; however, I'll choose the shortest one. To modify the abilities, I'll add a property for the current ability and make a different Monobehavior.
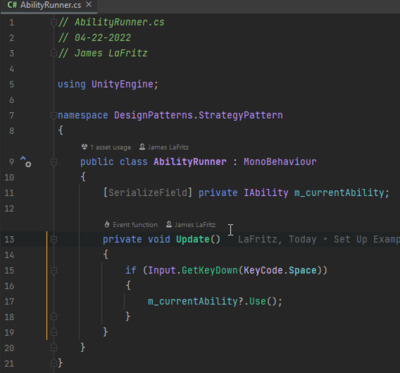
I can easily switch abilities now.
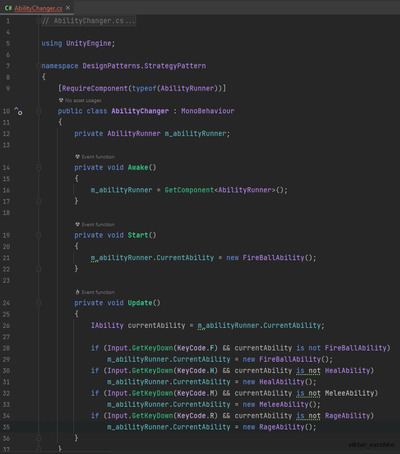
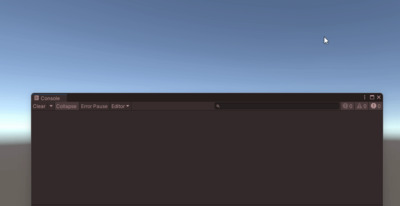
Conclusion
You should keep the Strategy Pattern in your toolkit. It enabled II to tidy up the code in my ability system and increase its reliance on SOILD. My code is now Open for extensions but Closed for changes. Additionally, it gives each function in my code a distinct duty. I can quickly include new, versatile talents into my system. All I have to do to alter how one ability functions is alter that one ability; I do not have to look for it in the switch statement. Additionally, altering how the abilities are utilized is simple. They are an Interface, which enables me to give everything a power. For instance, I could turn the Fireball into a MonoBehavior and prefab, or I could turn the Heal power into a Scriptable Object. The Melee Ability might be turned into a weapon. As long as people follow the interface, it doesn't matter.